The source code should be written by the language which is interpreted by the assembler or the compiler. When using MPASM, an assembly language is used. When you write a source code with the C language, the compiler of the C language is necessary.
The compiler interprets the sentence which was written in 1 line and spreads out to the instruction group(Generally, it is more than one instruction) to realize it. In case of the assembler, it changes each source code into the instruction. The C language can make a program with few source codes. But, a wasteful machine code is sometimes contained by the performance of the compiler. It is because it replaces with the machine code automatically.
When using a compiler, you can make a program easily. When using a memory efficiently, the assembler suits.
The source code file can be created using any ASCII text file editor. The lowercase and the uppercase are to be OK as the character.
I will explain the making of the source code which used an assembly language on these pages.
Source code format
The format of the source code is shown below. The maximum column width is 255 characters.
Label Mnemonic Operand ;Comment
;Comment
You can make 1 line only a comment, too. Of course, it is to be OK even if it doesn't write.
Generally, using the TAB, the position of the mnemonic, the operand, the comment is arranged for. It is for that it is easy to see.
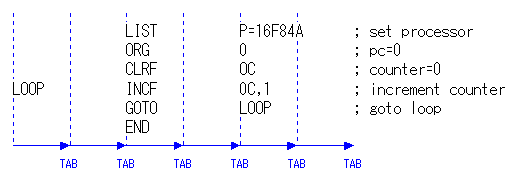
 | Labels |  | A label must start in colimn 1. |
 | It may be followed by a colon ( : ), space, tab or the end of line. |
 | Labels must begin with an alpha character or an under bar ( _ ) . |
 | Labels may contain alphanumeric characters, the under bar and question mark. |
 | Labels may be up to 32 characters long. |
 | It isn't possible to use the reserved word of the assembler for labels. |
| Because "B" becomes the reserved word which shows "Binary", it isn't possible to use for labels. |
 |
Mnemonics |  | Assembler instruction mnemonics, assembler directives and macro calls must begin in colum two or greater. |
 | If there is a label on the same line, instructions must be separated from that label by a colon, or by one more spaces or tabs. |
| Generally, the position of the instructions are arranged for using the tab. |
 | The character which it is possible to use is an alpha character or an under bar. |
 |
Operands |  | Operands must be separated from mnemonics by one more spaces, or tabs. |
 | Multiple operands must be separated by commas. |
| The spaces can be put behind the commas for that it is easy to see. |
 |
Comments |  | MPASM treats anything after a semicolon as a comment. |
 | All characters following the semicolon are ignored through the end of the line. |
 | String constants containing a semicolon are allowed and not confused with comments. |
Directive Language
PIC16F84A has 35 kinds of instructions. These instructions are the instructions to use when PIC processes.
MPASM has the directives to control the operation of the assembler as well as it. MPASM has 58 kinds of directives.
These directives are used according to need. However, in the end of the source code, the END must be always written.
When there is not an END, the assembler doesn't recognize written contents as the source code.
I will explain directives which seems to be often used below.
[ ] | : | Optional Arguments |  | expr | : | Expression |
< > | : | Variables. Text you supply |
|  | : | Space |
| | : | An OR selection |
|
|
|
|
 |
_ _CONFIG | Set Processor Configuration Bits |
Syntax | _ _CONFIG <expr> |
Descripton |
Sets the processor's configuration bits to the value described by <expr>.
Before this directive is used, the processor must be declared through the command line, the LIST directive, or the PROCESSOR directive. |
|
 |
DB | Declare Data of One Byte |
Syntax | [<label>] DB <expr>[,<expr>,...,<expr>] |
Descripton | Reserve program memory words with packed 8-bit values. |
|
 |
DT | Define Table |
Syntax | [<label>] DT <expr>[,<expr>,...,<expr>] |
Descripton | Generates a series of RETLW instructions, one instruction for each <expr>. |
|
 |
EQU | Define an Assembler Constant |
Syntax | <label> EQU <expr> |
Descripton | The value of <expr> is assigned to <label>. |
|
 |
END | End Program Block |
Syntax | END |
Descripton | Indicates the end of the program. |
|
 |
INCLUDE | Include Additional Source File |
Syntax | INCLUDE <<include_file>>|"<include_file>" |
Descripton | The specified file is read in as source code. |
|
 |
LIST | List Option |
Syntax | LIST [<list_option>,...,<list_option>] |
Descripton |
Occurring on a line by itself, the LIST directive has the effect of turning listing output on, if it had been previously turned off. Otherwise, one of the following list option can be supplied to control the assembly process or format the listing file.
Options | Default | Description |
b=nnn | 8 | Set tab spaces. |
c=nnn | 132 | Set column width. |
f=<format> | INHX8M | Set the hex file output. |
free | FIXED | Use free-format parser. |
fixed | FIXED | Use fixed-format parser. |
mm=ON|OFF | ON | Print memory map in list file. |
n=nnn | 60 | Set lines per page. |
p=<type> | None | Set processor type. |
r=<radix> | hex |
Set default radix.
hex, dec, oct |
st=ON|OFF | ON | Print symbol table in list file. |
t=ON|OFF | OFF | Truncate lines of listing. |
w=0|1|2 | 0 | Set the message level. |
x=ON|OFF | ON | Turn macro expansion on or off. |
All LIST options are evaluated as decimal numbers. |
|
 |
ORG | Set Program Origin |
Syntax | [label] ORG <expr> |
Descripton |
Set the program origin for subsequent code at the address defined in <expr>.
If no ORG is specified, code generation will begin at address zero. |
|
 |
PROCESSOR | Set Processor Type |
Syntax | PROCESSOR <processor_type> |
Descripton | Set the processor type to <processor_type>. |
|
 |
SET | Set an Assembler Variable |
Syntax | <label> SET <expr> |
Descripton | <label> is assigned the value of the valid MPASM expression specified by <expr>. The SET directive is functionally equivalent to the EQU directive except that SET value may be subsequently altered by other SET directives. |
|
 |
IF
ELSE
ENDIF |
Begin Conditional Assembled Code Block
Begin Alternative Assembly Block IF
End Conditional Assembly Block |
Syntax |
IF <expr>
........
ELSE
........
ENDIF |
Descripton |
Begin execution of a conditional assembly block. If <expr> evaluates to true, the code immadiately following the IF will assemble. Otherwise, subsequent code is skipped until an ELSE directive or an ENDIF directive is encountered.
An expression that evaluates to zero is considered logically FALSE. An expression that evaluates to any other value is considered logically TRUE. The IF and WHILE directives operate on the logical value of an expression. A relational TRUE expression is guaranteed to return a nonzero value, FALSE a value of zero.
These directives don't generate instructions like the C language. They only control the source code to assemble. |
|
 |
WHILE
ENDW |
Perform Loop While Condition is True
End a While Loop |
Syntax |
WHILE <expr>
........
ENDW |
Descripton |
The lines between the WHILE and the ENDW are assembled as long as <expr> evaluates to TRUE. An expression that evaluates to zero is considered logically FALSE. An expression that evaluates to any other value is considered logically TRUE. A relational TRUE expression is guaranteed to return a non-zero value; FALSE a value of zero.
A WHILE loop can contain at most 100 lines and be repeated a maximum of 256 times.
These directives don't generate instructions like the C language. They only control the source code to assemble. |
|
Numeric Constants and Radix
MPASM supports the following radix forms. The default radix is hexadecimal.
Constants can be optionally preceded by a plus or minus sign. if unsigned, the value is assumed to be positive.
Radix Specifications
Type | Syntax | Example |
Decimal | D'<digits>' or .(dot)<digits> | D'100' or .100 |
Hexadecimal | H'<hex_digits>' or 0x<hex_digits> or 0<hex_digits>H | H'A8' or 0xA8 or 0A8H |
Octal | O'<octal_digits> | O'567' |
Binary | B'<binary_digits> | B'011001' |
ASCII | A'<characters>' or '<characters>' | A'ABC' or 'ABC' |
Arithmatic Operators and Precedence
Operator | Example |
$ | Current/Return program counter | goto $+3 |
( | Left Parenthesis | (length+1)*256 |
) | Right Parenthesis |
* | Multiply | a*b |
/ | Divide | a/b |
+ | Add | a+b |
- | Subtract | a-b |
high | Return high byte | movlw high TABLE |
low | Return low byte | movlw low TABLE |
>= | Greater or equal | if a>=b |
> | Greater than | if a>b |
<= | Less or equal | if a<=b |
< | Less than | if a<b |
== | Equal to | if (a==b) |
!= | Not equal to | if (a!=b) |
& | Bitwise AND | flag=flag&CHECK_BIT |
| | Bitwise inclusive OR | flag=flag|CHECK_BIT |
&& | Logical AND | if (a==b)&&(c==d) |
|| | Logical OR | if(a==b)||(c==d) |
! | Item NOT (logical complement) | if !(a==b) |
|